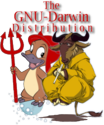
|
Basic NodeVisitor implementation for rendering a scene.
Inheritance:
Public Methods-
CullVisitor()
-
virtual ~CullVisitor()
-
virtual CullVisitor* cloneType() const
-
virtual void reset()
-
virtual osg::Vec3 getEyePoint() const
-
virtual float getDistanceToEyePoint(const osg::Vec3& pos, bool withLODScale) const
-
virtual float getDistanceFromEyePoint(const osg::Vec3& pos, bool withLODScale) const
-
virtual void apply(osg::Node&)
-
virtual void apply(osg::Geode& node)
-
virtual void apply(osg::Billboard& node)
-
virtual void apply(osg::LightSource& node)
-
virtual void apply(osg::ClipNode& node)
-
virtual void apply(osg::Group& node)
-
virtual void apply(osg::Transform& node)
-
virtual void apply(osg::Projection& node)
-
virtual void apply(osg::Switch& node)
-
virtual void apply(osg::LOD& node)
-
virtual void apply(osg::ClearNode& node)
-
virtual void apply(osg::OccluderNode& node)
-
virtual void apply(osg::Impostor& node)
-
void setClearNode(const osg::ClearNode* earthSky)
-
const osg::ClearNode* getClearNode() const
-
void setImpostorsActive(bool active)
- Switch the creation of Impostors on or off.
-
bool getImpostorsActive() const
- Get whether impostors are active or not.
-
void setImpostorPixelErrorThreshold(float numPixels)
- Set the impostor error threshold.
-
float getImpostorPixelErrorThreshold() const
- Get the impostor error threshold
-
void setDepthSortImpostorSprites(bool doDepthSort)
- Set whether ImpsotorSprite's should be placed in a depth sorted bin for rendering
-
bool setDepthSortImpostorSprites() const
- Get whether ImpsotorSprite's are depth sorted bin for rendering
-
void setNumberOfFrameToKeepImpostorSprites(int numFrames)
- Set the number of frames that an ImpsotorSprite's is kept whilst not being beyond, before being recycled
-
int getNumberOfFrameToKeepImpostorSprites() const
- Get the number of frames that an ImpsotorSprite's is kept whilst not being beyond, before being recycled
-
void setComputeNearFarMode(ComputeNearFarMode cnfm)
-
ComputeNearFarMode getComputeNearFarMode() const
-
inline void pushStateSet(const osg::StateSet* ss)
- Push state set on the current state group.
-
inline void popStateSet()
- Pop the top state set and hence associated state group.
-
inline void setRenderGraph(RenderGraph* rg)
-
inline RenderGraph* getRootRenderGraph()
-
inline RenderGraph* getCurrentRenderGraph()
-
inline void setRenderStage(RenderStage* rg)
-
inline RenderStage* getRenderStage()
-
inline RenderBin* getCurrentRenderBin()
-
inline void setCurrentRenderBin(RenderBin* rb)
-
inline float getCalculatedNearPlane() const
-
inline float getCalculatedFarPlane() const
-
void updateCalculatedNearFar(const osg::Matrix& matrix, const osg::Drawable& drawable)
-
void updateCalculatedNearFar(const osg::Matrix& matrix, const osg::BoundingBox& bb)
-
void updateCalculatedNearFar(const osg::Vec3& pos)
-
virtual void popProjectionMatrix()
- reimplement CullStack's popProjectionMatrix() adding clamping of the projection matrix to the computed near and far
-
void setState(osg::State* state)
-
osg::State* getState()
-
const osg::State* getState() const
-
inline void addDrawable(osg::Drawable* drawable, osg::RefMatrix* matrix)
- Add a drawable to current render graph
-
inline void addDrawableAndDepth(osg::Drawable* drawable, osg::RefMatrix* matrix, float depth)
- Add a drawable and depth to current render graphAdd a drawable and depth to current render graph
-
inline void addPositionedAttribute(osg::RefMatrix* matrix, const osg::StateAttribute* attr)
- Add an attribute which is positioned related to the modelview matrixAdd an attribute which is positioned related to the modelview matrix
-
inline RenderLeaf* createOrReuseRenderLeaf(osg::Drawable* drawable, osg::RefMatrix* projection, osg::RefMatrix* matrix, float depth)
Public Members-
enum ComputeNearFarMode
Protected Fields-
osg::ref_ptr<RenderGraph> _rootRenderGraph
-
RenderGraph* _currentRenderGraph
-
osg::ref_ptr<RenderStage> _rootRenderStage
-
RenderBin* _currentRenderBin
-
ComputeNearFarMode _computeNearFar
-
float _computed_znear
-
float _computed_zfar
-
osg::ref_ptr<const osg::ClearNode> _clearNode
-
bool _impostorActive
-
bool _depthSortImpostorSprites
-
float _impostorPixelErrorThreshold
-
int _numFramesToKeepImpostorSprites
-
RenderLeafList _reuseRenderLeafList
-
unsigned int _currentReuseRenderLeafIndex
-
osg::ref_ptr<osg::ImpostorSpriteManager> _impostorSpriteManager
-
osg::ref_ptr<osg::State> _state
Protected Methods-
CullVisitor(const CullVisitor&)
- prevent unwanted copy construction
-
CullVisitor& operator = (const CullVisitor&)
- prevent unwanted copy operator
-
inline void handle_cull_callbacks_and_traverse(osg::Node& node)
-
inline void handle_cull_callbacks_and_accept(osg::Node& node, osg::Node* acceptNode)
-
osg::ImpostorSprite* createImpostorSprite(osg::Impostor& node)
- create an impostor sprite by setting up a pre-rendering stage to generate the impostor texture.
Protected Members-
typedef std::vector< osg::ref_ptr<RenderLeaf> > RenderLeafList
Documentation
Basic NodeVisitor implementation for rendering a scene.
This visitor traverses the scene graph, collecting transparent and
opaque osg::Drawables into a depth sorted transparent bin and a state
sorted opaque bin. The opaque bin is rendered first, and then the
transparent bin in rendered in order from the furthest osg::Drawable
from the eye to the one nearest the eye.
CullVisitor()
virtual ~CullVisitor()
virtual CullVisitor* cloneType() const
virtual void reset()
virtual osg::Vec3 getEyePoint() const
virtual float getDistanceToEyePoint(const osg::Vec3& pos, bool withLODScale) const
virtual float getDistanceFromEyePoint(const osg::Vec3& pos, bool withLODScale) const
virtual void apply(osg::Node&)
virtual void apply(osg::Geode& node)
virtual void apply(osg::Billboard& node)
virtual void apply(osg::LightSource& node)
virtual void apply(osg::ClipNode& node)
virtual void apply(osg::Group& node)
virtual void apply(osg::Transform& node)
virtual void apply(osg::Projection& node)
virtual void apply(osg::Switch& node)
virtual void apply(osg::LOD& node)
virtual void apply(osg::ClearNode& node)
virtual void apply(osg::OccluderNode& node)
virtual void apply(osg::Impostor& node)
void setClearNode(const osg::ClearNode* earthSky)
const osg::ClearNode* getClearNode() const
void setImpostorsActive(bool active)
- Switch the creation of Impostors on or off.
Setting active to false forces the CullVisitor to use the Impostor
LOD children for rendering. Setting active to true forces the
CullVisitor to create the appropriate pre-rendering stages which
render to the ImpostorSprite's texture.
bool getImpostorsActive() const
- Get whether impostors are active or not.
void setImpostorPixelErrorThreshold(float numPixels)
- Set the impostor error threshold.
Used in calculation of whether impostors remain valid.
float getImpostorPixelErrorThreshold() const
- Get the impostor error threshold
void setDepthSortImpostorSprites(bool doDepthSort)
- Set whether ImpsotorSprite's should be placed in a depth sorted bin for rendering
bool setDepthSortImpostorSprites() const
- Get whether ImpsotorSprite's are depth sorted bin for rendering
void setNumberOfFrameToKeepImpostorSprites(int numFrames)
- Set the number of frames that an ImpsotorSprite's is kept whilst not being beyond,
before being recycled
int getNumberOfFrameToKeepImpostorSprites() const
- Get the number of frames that an ImpsotorSprite's is kept whilst not being beyond,
before being recycled
enum ComputeNearFarMode
DO_NOT_COMPUTE_NEAR_FAR
COMPUTE_NEAR_FAR_USING_BOUNDING_VOLUMES
COMPUTE_NEAR_FAR_USING_PRIMITIVES
void setComputeNearFarMode(ComputeNearFarMode cnfm)
ComputeNearFarMode getComputeNearFarMode() const
inline void pushStateSet(const osg::StateSet* ss)
- Push state set on the current state group.
If the state exists in a child state group of the current
state group then move the current state group to that child.
Otherwise, create a new state group for the state set, add
it to the current state group then move the current state
group pointer to the new state group.
inline void popStateSet()
- Pop the top state set and hence associated state group.
Move the current state group to the parent of the popped
state group.
inline void setRenderGraph(RenderGraph* rg)
inline RenderGraph* getRootRenderGraph()
inline RenderGraph* getCurrentRenderGraph()
inline void setRenderStage(RenderStage* rg)
inline RenderStage* getRenderStage()
inline RenderBin* getCurrentRenderBin()
inline void setCurrentRenderBin(RenderBin* rb)
inline float getCalculatedNearPlane() const
inline float getCalculatedFarPlane() const
void updateCalculatedNearFar(const osg::Matrix& matrix, const osg::Drawable& drawable)
void updateCalculatedNearFar(const osg::Matrix& matrix, const osg::BoundingBox& bb)
void updateCalculatedNearFar(const osg::Vec3& pos)
virtual void popProjectionMatrix()
- reimplement CullStack's popProjectionMatrix() adding clamping of the projection matrix to the computed near and far
void setState(osg::State* state)
osg::State* getState()
const osg::State* getState() const
CullVisitor(const CullVisitor&)
- prevent unwanted copy construction
CullVisitor& operator = (const CullVisitor&)
- prevent unwanted copy operator
inline void handle_cull_callbacks_and_traverse(osg::Node& node)
inline void handle_cull_callbacks_and_accept(osg::Node& node, osg::Node* acceptNode)
osg::ImpostorSprite* createImpostorSprite(osg::Impostor& node)
- create an impostor sprite by setting up a pre-rendering stage
to generate the impostor texture.
osg::ref_ptr<RenderGraph> _rootRenderGraph
RenderGraph* _currentRenderGraph
osg::ref_ptr<RenderStage> _rootRenderStage
RenderBin* _currentRenderBin
ComputeNearFarMode _computeNearFar
float _computed_znear
float _computed_zfar
osg::ref_ptr<const osg::ClearNode> _clearNode
bool _impostorActive
bool _depthSortImpostorSprites
float _impostorPixelErrorThreshold
int _numFramesToKeepImpostorSprites
typedef std::vector< osg::ref_ptr<RenderLeaf> > RenderLeafList
RenderLeafList _reuseRenderLeafList
unsigned int _currentReuseRenderLeafIndex
osg::ref_ptr<osg::ImpostorSpriteManager> _impostorSpriteManager
osg::ref_ptr<osg::State> _state
inline void addDrawable(osg::Drawable* drawable, osg::RefMatrix* matrix)
- Add a drawable to current render graph
inline void addDrawableAndDepth(osg::Drawable* drawable, osg::RefMatrix* matrix, float depth)
- Add a drawable and depth to current render graphAdd a drawable and depth to current render graph
inline void addPositionedAttribute(osg::RefMatrix* matrix, const osg::StateAttribute* attr)
- Add an attribute which is positioned related to the modelview matrixAdd an attribute which is positioned related to the modelview matrix
inline RenderLeaf* createOrReuseRenderLeaf(osg::Drawable* drawable, osg::RefMatrix* projection, osg::RefMatrix* matrix, float depth)
- This class has no child classes.
Alphabetic index HTML hierarchy of classes or Java
This page was generated with the help of DOC++.
|